React Hooks revolutionized the way we write functional components in React, offering a powerful and concise way to manage state, side effects, and other functionalities previously handled by class components. This blog post dives deep into the world of React Hooks, explaining their core concepts, different types, and best practices for effective use in your projects.
What are React Hooks?
Introduced in React 16.8, Hooks are functions that let you “hook into” React features like state and lifecycle methods from functional components. Unlike class components with their built-in state management and lifecycle methods, functional components traditionally lacked these functionalities. Hooks bridge this gap, allowing you to write cleaner, more maintainable, and often more performant code.
Core Rules of Using React Hooks:
While Hooks offer flexibility, there are a few essential rules to follow:
- Hooks Can Only Be Called at the Top Level: You can only call Hooks at the top level of a functional component, before the return This ensures they run in the correct order and prevents unexpected behavior.
- Hooks Can’t Be Called Conditionally: Hooks rely on React’s rendering order. Calling them conditionally within loops, if statements, or other conditional logic can lead to unpredictable results.
- Hooks Can Use Other Hooks: While Hooks should be called at the top level, they can freely call other Hooks within their definition. This allows you to build more complex functionalities by composing Hooks together.
Essential Hooks for Every React Developer:
Here’s a breakdown of some of the most commonly used Hooks in React projects:
- useState Hook: The useState Hook is the foundation for state management in functional components. It allows you to declare state variables and a function to update them. This replaces the need for the this.state and setState methods in class components.
JavaScript
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
- useEffect Hook: The useEffect Hook allows you to perform side effects in your functional components. These side effects can include data fetching, subscriptions, or any operation that interacts with the outside world. You can optionally provide a dependency array to control when the effect runs.
JavaScript
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch(‘https://api.example.com/users’)
.then(response => response.json())
.then(data => setUsers(data));
}, []); // Empty dependency array ensures the effect runs only once on component mount
return (
<ul>
{users.map(user => (
<li key={user.id}<{user.name}</li>
))}
</ul>
);
}
- useContext Hook: The useContext Hook allows you to share data across components without explicitly passing props through every level of the component hierarchy. It’s particularly useful for managing global application state.
JavaScript
const MyContext = React.createContext();
function App() {
const [theme, setTheme] = useState(‘light’);
return (
<MyContext.Provider value={{ theme, setTheme }}>
<Content />
</MyContext.Provider>
);
}
function Content() {
const context = useContext(MyContext);
return (
<div style={{ backgroundColor: context.theme }}>
<Settings theme={context.theme} setTheme={context.setTheme} />
</div>
);
}
function Settings({ theme, setTheme }) {
const toggleTheme = () => setTheme(theme === ‘light’ ? ‘dark’ : ‘light’);
return (
<button onClick={toggleTheme}>Toggle Theme ({theme})</button>
);
}
JavaScript
- useMemo Hook: The useMemo Hook allows you to memoize expensive calculations or derived data within your components. This helps improve performance by avoiding unnecessary re-calculations as long as the dependencies haven’t changed.
JavaScript
function ProductList({ products, discountPercentage }) {
const getDiscountedPrice = React.useMemo(() => {
return (product) => product.price * (1 – discountPercentage);
}, [discountPercentage]);
return (
<ul>
{products.map((product) => (
<li key={product.id}>
{product.name} – Discounted Price: ${getDiscountedPrice(product)}
</li>
))}
</ul>
);
}
In this example:
- The getDiscountedPrice function calculates the discounted price for a product.
- The function is memoized using useMemo.
- The dependency array includes only discountPercentage. This means the function will be re-calculated only when the discount percentage changes, not for every product in the list.
Want to build a cutting-edge application with React? Get in touch with our team now!
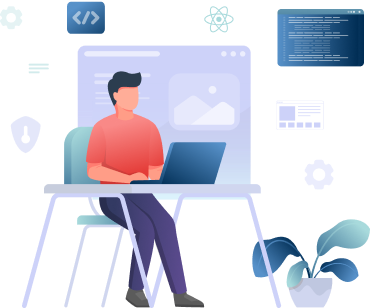